- #typescript
- /
- #react js
- /
- #webassembly
- /
- #rust
- /
React and WebAssembly: Unlocking High-Performance Apps
In this post, we’ll explore what WebAssembly is, why you’d want to use it with React, and how to get started.
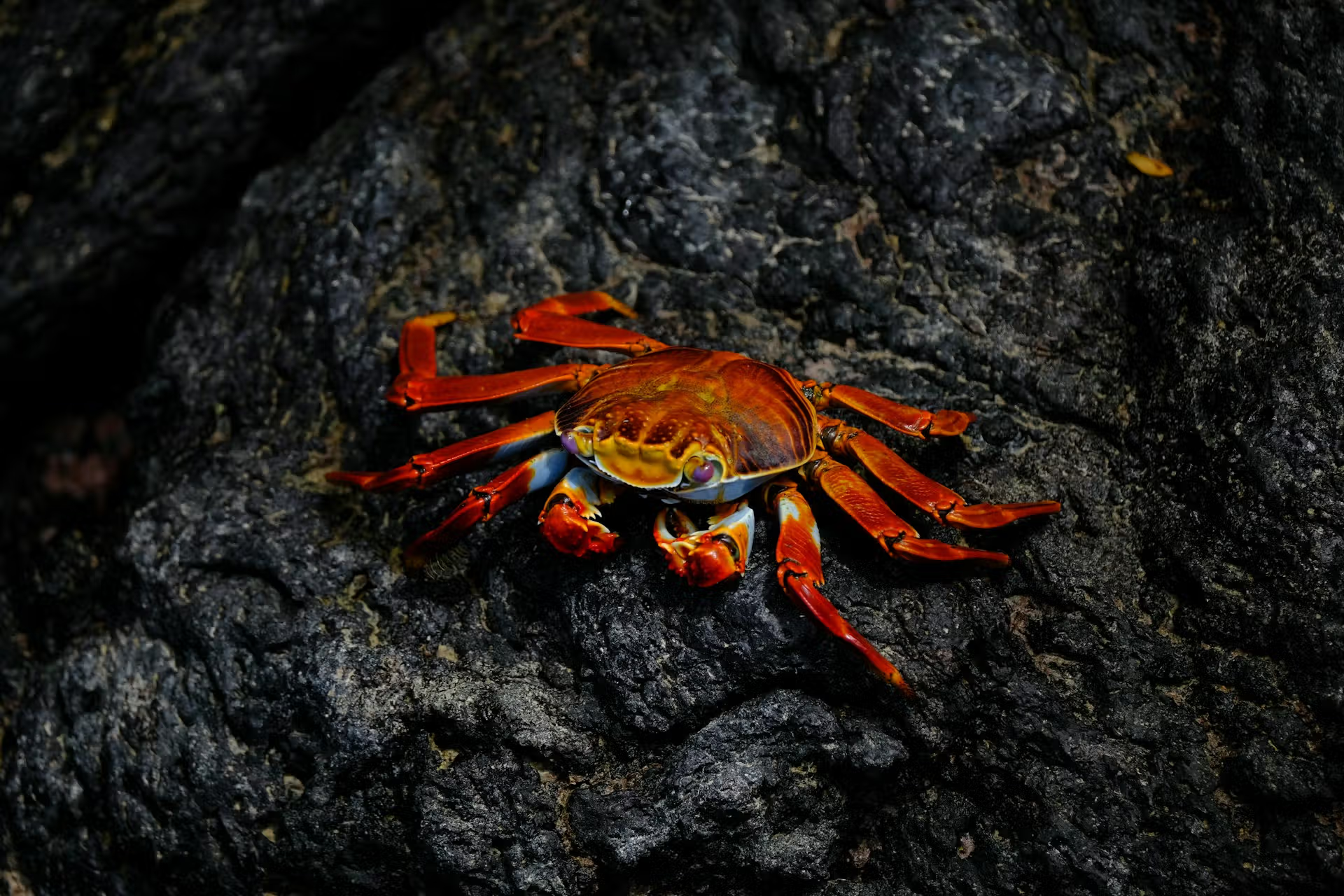
Web performance is more important than ever. With applications becoming more complex, developers are constantly looking for ways to optimize performance. One of the most exciting advancements in web development is WebAssembly (WASM) — a low-level, binary instruction format that runs in the browser at near-native speed. When combined with React, WebAssembly opens up new possibilities for building high-performance web applications.
🚀 What is WebAssembly (WASM)?
WebAssembly (WASM) is a portable binary format that allows code written in languages like C, C++, Rust, and others to run in the browser at native speed. It was designed to complement JavaScript, enabling performance-critical parts of your application to be written in more efficient languages while still integrating seamlessly with the web ecosystem.
Key Features of WebAssembly
- High Performance: Near-native execution speed.
- Portability: Runs on all major browsers and platforms.
- Interoperability: Works alongside JavaScript.
- Security: Sandboxed environment for safe execution.
Why Use WebAssembly with React?
React is already a performant library for building user interfaces, but for CPU-intensive tasks, such as real-time data processing, image manipulation, or 3D rendering, JavaScript may hit its limits. This is where WebAssembly shines.
Use Cases for React + WebAssembly
- Real-time Data Visualization: Handling large datasets or complex calculations without blocking the UI.
- Multimedia Processing: Image, audio, and video manipulation.
- Gaming and 3D Graphics: Powering graphics-heavy applications using WebGL.
- Cryptography and Compression: High-performance encryption, compression, and decompression in the browser.
- Machine Learning: Running inference for trained ML models in the browser.
Setting Up WebAssembly with React
Let’s walk through a simple example of integrating WebAssembly in a React app. For this demo, we’ll use Rust to write a WebAssembly module.
Step 1: Install Rust and wasm-pack
First, install Rust and the wasm-pack
tool, which helps compile Rust into WebAssembly.
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
cargo install wasm-pack
Step 2: Create a Rust Library
Create a new Rust project and add a function to calculate the Fibonacci sequence (a CPU-heavy task).Create a new Rust project and add a function to calculate the Fibonacci sequence (a CPU-heavy task).
// src/lib.rs
#[no_mangle]
pub fn fibonacci(n: u32) -> u32 {
match n {
0 => 0,
1 => 1,
_ => fibonacci(n - 1) + fibonacci(n - 2),
}
}
Step 3: Compile to WebAssembly
Use wasm-pack
to compile the Rust project to WebAssembly.
wasm-pack build --target web
Step 4: Integrate WebAssembly in React
In your React app, import and use the generated WebAssembly module.
import React, { useState } from 'react';
function App() {
const [fibResult, setFibResult] = useState<number | null>(null);
const calculateFibonacci = async (n: number) => {
const wasm = await import('./wasm_module'); // Import your Wasm module
const result = wasm.fibonacci(n);
setFibResult(result);
};
return (
<div>
<h1>React + WebAssembly Example</h1>
<input
type="number"
placeholder="Enter a number"
onChange={(e) => calculateFibonacci(Number(e.target.value))}
/>
{fibResult !== null && <p>Fibonacci Result: {fibResult}</p>}
</div>
);
}
export default App;
Performance Gains: What to Expect
By offloading computationally intensive tasks to WebAssembly, you can reduce UI blocking and improve the responsiveness of your React app. However, WebAssembly isn’t always the best solution for every problem. Use it when raw performance is essential, and stick to JavaScript for simpler tasks to avoid unnecessary complexity.
Best Practices for React and WebAssembly Integration
- Benchmark First: Ensure that the performance gains justify the added complexity.
- Avoid Over-Optimization: Not everything needs to be written in WebAssembly. Use it where it makes the most impact.
- Memory Management: Be mindful of WebAssembly’s memory model. Share data between JavaScript and WASM efficiently.
- Error Handling: WASM debugging can be tricky. Use tools like
wasm-bindgen
andconsole.error
for better error tracking.
The Future of React and WebAssembly
As WebAssembly continues to evolve (with features like garbage collection and threading on the horizon), it’s poised to play a significant role in the future of web development. When combined with React, it opens up new doors for high-performance, interactive web applications that were previously impossible to build with pure JavaScript. Tools like Figma, AutoDesk and Tensorflow.js already leverage WASM, and list is just gonna keep growing.
Want to go deeper? Let me know if you’d like a follow-up post on optimizing WebAssembly memory or integrating WebAssembly with other frameworks like Next.js!